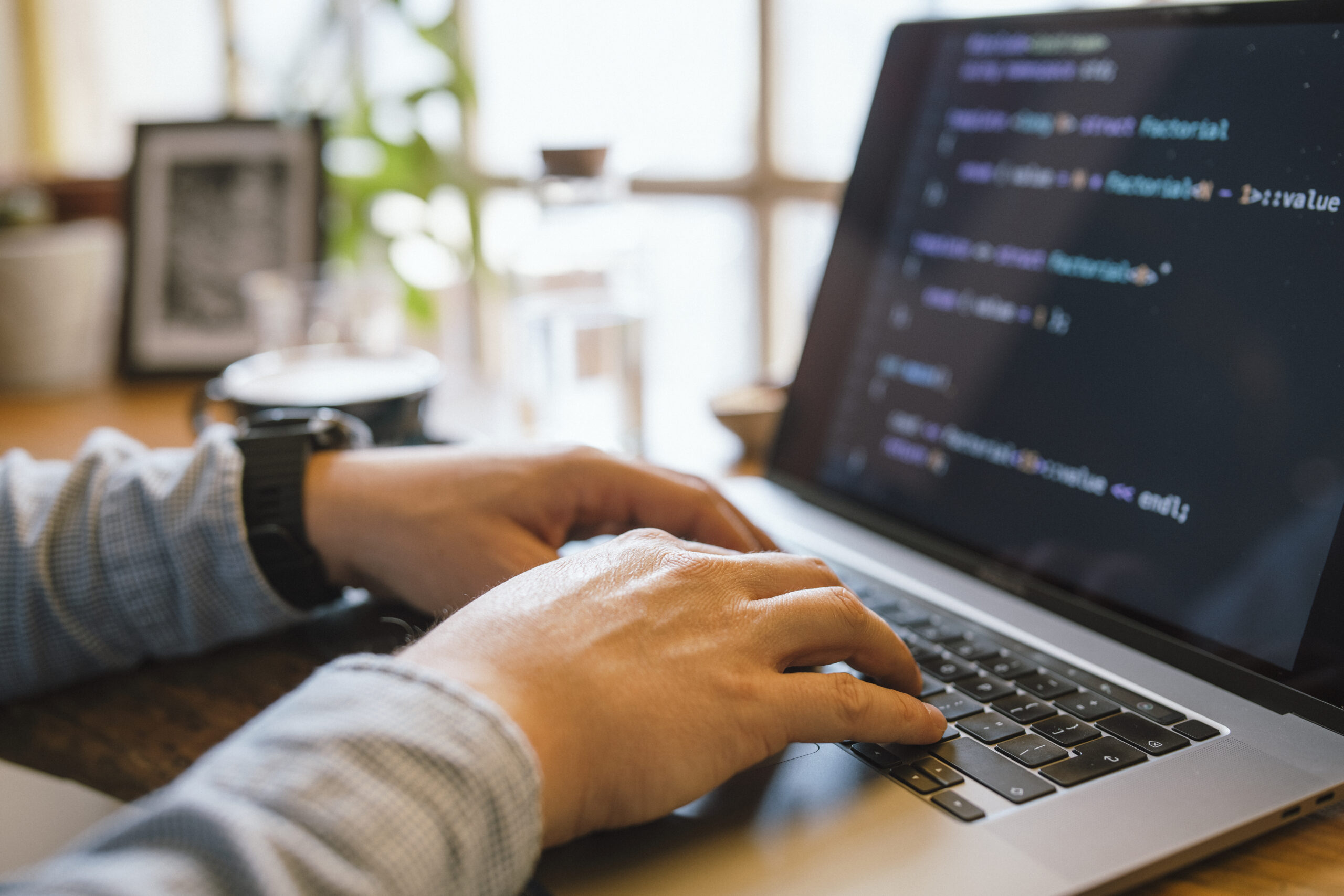
Debugging is One of the more critical — however typically missed — abilities within a developer’s toolkit. It's actually not nearly repairing damaged code; it’s about comprehending how and why items go Mistaken, and Mastering to Imagine methodically to unravel complications efficiently. No matter whether you're a novice or possibly a seasoned developer, sharpening your debugging techniques can help you save several hours of irritation and significantly boost your productivity. Listed here are a number of methods to assist developers amount up their debugging activity by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest means builders can elevate their debugging techniques is by mastering the instruments they use on a daily basis. When composing code is a single Section of enhancement, understanding how you can communicate with it effectively during execution is Similarly vital. Modern-day growth environments come Geared up with strong debugging capabilities — but many builders only scratch the surface area of what these tools can perform.
Consider, for instance, an Built-in Growth Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment allow you to established breakpoints, inspect the value of variables at runtime, action by means of code line by line, and even modify code to the fly. When employed effectively, they Allow you to notice specifically how your code behaves during execution, that's invaluable for tracking down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for entrance-finish builders. They permit you to inspect the DOM, watch network requests, look at real-time functionality metrics, and debug JavaScript inside the browser. Mastering the console, resources, and community tabs can change disheartening UI problems into workable responsibilities.
For backend or method-stage builders, tools like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate more than managing procedures and memory management. Understanding these instruments may have a steeper Understanding curve but pays off when debugging effectiveness challenges, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be relaxed with Variation control methods like Git to grasp code heritage, find the exact second bugs have been launched, and isolate problematic variations.
Ultimately, mastering your resources implies going past default options and shortcuts — it’s about establishing an personal familiarity with your enhancement environment to ensure that when problems come up, you’re not misplaced at nighttime. The higher you recognize your equipment, the more time it is possible to commit fixing the actual difficulty as an alternative to fumbling by way of the method.
Reproduce the challenge
The most vital — and sometimes ignored — actions in helpful debugging is reproducing the condition. In advance of leaping to the code or producing guesses, developers need to produce a reliable natural environment or situation exactly where the bug reliably appears. Without the need of reproducibility, repairing a bug turns into a sport of chance, generally resulting in wasted time and fragile code variations.
Step one in reproducing an issue is accumulating just as much context as you possibly can. Ask thoughts like: What steps brought about the issue? Which ecosystem was it in — growth, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you might have, the simpler it results in being to isolate the exact conditions underneath which the bug occurs.
When you finally’ve collected plenty of details, try to recreate the situation in your local natural environment. This might necessarily mean inputting the exact same info, simulating identical consumer interactions, or mimicking system states. If The problem seems intermittently, contemplate producing automated assessments that replicate the edge cases or condition transitions involved. These checks not simply aid expose the problem and also prevent regressions Sooner or later.
Occasionally, The difficulty could be environment-distinct — it might take place only on sure working devices, browsers, or less than distinct configurations. Applying resources like virtual equipment, containerization (e.g., Docker), or cross-browser testing platforms may be instrumental in replicating these kinds of bugs.
Reproducing the situation isn’t just a step — it’s a frame of mind. It involves tolerance, observation, and a methodical strategy. But when you finally can consistently recreate the bug, you are previously midway to correcting it. Which has a reproducible state of affairs, You may use your debugging tools much more efficiently, examination likely fixes safely and securely, and converse additional Evidently with all your workforce or users. It turns an abstract complaint right into a concrete obstacle — Which’s in which developers thrive.
Read through and Fully grasp the Mistake Messages
Mistake messages in many cases are the most worthy clues a developer has when a little something goes wrong. Instead of observing them as discouraging interruptions, builders should really learn to take care of mistake messages as immediate communications from the system. They frequently tell you exactly what transpired, where by it took place, and often even why it occurred — if you know how to interpret them.
Start by examining the information thoroughly As well as in whole. A lot of developers, especially when less than time force, glance at the very first line and immediately get started building assumptions. But deeper in the mistake stack or logs could lie the accurate root induce. Don’t just duplicate and paste error messages into search engines like google — browse and fully grasp them very first.
Crack the error down into parts. Can it be a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a particular file and line selection? What module or function triggered it? These issues can information your investigation and stage you towards the responsible code.
It’s also handy to know the terminology with the programming language or framework you’re utilizing. Mistake messages in languages like Python, JavaScript, or Java frequently observe predictable patterns, and Mastering to recognize these can substantially speed up your debugging approach.
Some faults are vague or generic, As well as in those situations, it’s crucial to examine the context where the error transpired. Check out related log entries, input values, and up to date changes inside the codebase.
Don’t forget compiler or linter warnings either. These generally precede larger problems and provide hints about probable bugs.
Finally, error messages usually are not your enemies—they’re your guides. Finding out to interpret them the right way turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a more effective and assured developer.
Use Logging Correctly
Logging is One of the more powerful resources within a developer’s debugging toolkit. When applied correctly, it offers serious-time insights into how an software behaves, helping you understand what’s happening website under the hood without needing to pause execution or step with the code line by line.
A superb logging approach commences with realizing what to log and at what degree. Frequent logging stages incorporate DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for in-depth diagnostic information and facts all through enhancement, Facts for normal functions (like profitable start off-ups), Alert for opportunity difficulties that don’t split the application, ERROR for precise challenges, and Deadly once the method can’t continue.
Stay clear of flooding your logs with too much or irrelevant knowledge. A lot of logging can obscure essential messages and decelerate your process. Target important functions, condition alterations, input/output values, and critical choice details with your code.
Format your log messages Evidently and persistently. Consist of context, such as timestamps, ask for IDs, and function names, so it’s simpler to trace problems in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
Throughout debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without halting the program. They’re especially precious in manufacturing environments where by stepping by way of code isn’t possible.
Moreover, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, wise logging is about stability and clarity. With a properly-thought-out logging tactic, you'll be able to lessen the time it takes to spot difficulties, gain deeper visibility into your purposes, and improve the All round maintainability and trustworthiness of one's code.
Consider Similar to a Detective
Debugging is not just a specialized endeavor—it's a type of investigation. To properly detect and repair bugs, developers have to solution the process like a detective fixing a thriller. This way of thinking allows break down sophisticated troubles into workable pieces and follow clues logically to uncover the root trigger.
Start out by accumulating proof. Think about the symptoms of the issue: error messages, incorrect output, or functionality difficulties. The same as a detective surveys against the law scene, obtain just as much relevant information as you are able to without having jumping to conclusions. Use logs, check circumstances, and user reviews to piece collectively a clear image of what’s happening.
Subsequent, type hypotheses. Inquire your self: What might be creating this behavior? Have any modifications recently been made into the codebase? Has this difficulty happened in advance of beneath equivalent situations? The objective should be to slender down opportunities and discover prospective culprits.
Then, check your theories systematically. Try to recreate the condition in a very controlled environment. For those who suspect a certain perform or ingredient, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, request your code concerns and Enable the outcome lead you nearer to the truth.
Pay back near attention to smaller specifics. Bugs often disguise inside the the very least anticipated places—just like a missing semicolon, an off-by-just one error, or maybe a race problem. Be complete and individual, resisting the urge to patch The difficulty without having fully being familiar with it. Short term fixes may conceal the actual difficulty, just for it to resurface later.
And lastly, maintain notes on That which you attempted and figured out. Just as detectives log their investigations, documenting your debugging approach can save time for foreseeable future issues and support others recognize your reasoning.
By wondering like a detective, developers can sharpen their analytical competencies, tactic problems methodically, and turn into more effective at uncovering hidden difficulties in complex techniques.
Generate Exams
Composing checks is one of the most effective strategies to help your debugging skills and All round progress performance. Checks not only aid catch bugs early and also function a security Web that offers you self-confidence when producing alterations towards your codebase. A well-tested application is simpler to debug as it helps you to pinpoint precisely in which and when a difficulty happens.
Begin with unit exams, which give attention to specific features or modules. These modest, isolated assessments can speedily expose no matter if a certain bit of logic is Doing work as predicted. Each time a examination fails, you right away know in which to appear, considerably decreasing the time spent debugging. Device assessments are Specially beneficial for catching regression bugs—concerns that reappear following previously remaining preset.
Upcoming, integrate integration tests and close-to-conclusion exams into your workflow. These assist ensure that many areas of your application work alongside one another efficiently. They’re especially useful for catching bugs that come about in intricate methods with various elements or solutions interacting. If a little something breaks, your assessments can let you know which part of the pipeline unsuccessful and under what ailments.
Composing tests also forces you to definitely think critically regarding your code. To test a element appropriately, you need to be aware of its inputs, anticipated outputs, and edge cases. This volume of being familiar with Obviously prospects to raised code construction and much less bugs.
When debugging a problem, writing a failing take a look at that reproduces the bug could be a robust initial step. When the check fails constantly, you can center on repairing the bug and enjoy your test move when The difficulty is resolved. This strategy makes certain that the same bug doesn’t return Later on.
Briefly, writing exams turns debugging from a discouraging guessing game into a structured and predictable approach—encouraging you catch a lot more bugs, speedier plus more reliably.
Consider Breaks
When debugging a difficult situation, it’s quick to be immersed in the issue—watching your display screen for several hours, seeking solution following Remedy. But The most underrated debugging instruments is solely stepping absent. Having breaks helps you reset your mind, decrease disappointment, and sometimes see The problem from a new viewpoint.
When you're as well close to the code for also prolonged, cognitive fatigue sets in. You could commence overlooking clear problems or misreading code which you wrote just hrs earlier. Within this state, your Mind results in being fewer economical at challenge-resolving. A short walk, a coffee crack, or maybe switching to a unique process for 10–15 minutes can refresh your focus. Lots of builders report locating the root of a problem when they've taken time and energy to disconnect, allowing their subconscious function in the history.
Breaks also support avoid burnout, Particularly during for a longer period debugging periods. Sitting before a display, mentally stuck, is don't just unproductive and also draining. Stepping away helps you to return with renewed Electricity plus a clearer state of mind. You might quickly recognize a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a 5–ten minute crack. Use that time to maneuver around, extend, or do something unrelated to code. It could feel counterintuitive, Specially under restricted deadlines, but it in fact leads to more rapidly and more practical debugging Over time.
To put it briefly, taking breaks will not be an indication of weakness—it’s a wise strategy. It provides your Mind House to breathe, improves your point of view, and helps you stay away from the tunnel eyesight That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of solving it.
Master From Every Bug
Every single bug you come upon is more than just A brief setback—It is really an opportunity to increase to be a developer. Whether or not it’s a syntax mistake, a logic flaw, or maybe a deep architectural problem, each can instruct you something worthwhile for those who make an effort to mirror and assess what went wrong.
Begin by asking by yourself a handful of key concerns after the bug is settled: What induced it? Why did it go unnoticed? Could it are actually caught before with improved practices like device tests, code reviews, or logging? The answers usually reveal blind spots within your workflow or knowing and allow you to Create more robust coding practices relocating forward.
Documenting bugs may also be a superb behavior. Preserve a developer journal or sustain a log where you Be aware down bugs you’ve encountered, how you solved them, and what you acquired. Eventually, you’ll begin to see styles—recurring difficulties or widespread problems—which you could proactively stay away from.
In crew environments, sharing Everything you've discovered from the bug with the friends could be Particularly powerful. Whether it’s via a Slack concept, a short generate-up, or A fast understanding-sharing session, helping Many others stay away from the exact same difficulty boosts crew efficiency and cultivates a much better Understanding culture.
Additional importantly, viewing bugs as lessons shifts your mentality from stress to curiosity. As opposed to dreading bugs, you’ll start appreciating them as necessary elements of your enhancement journey. All things considered, some of the most effective developers are certainly not the ones who generate best code, but those who repeatedly discover from their faults.
In the end, Just about every bug you repair provides a brand new layer towards your skill established. So subsequent time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, much more able developer because of it.
Conclusion
Increasing your debugging skills normally takes time, observe, and patience — even so the payoff is large. It helps make you a far more economical, confident, and capable developer. The subsequent time you might be knee-deep in a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be much better at Whatever you do.